Implementing Gesture Activated Camera Using ATMEL SAM V71
Project Overview
My project proposal aimed to develop a gesture-activated camera, enabling users to trigger various camera commands through a gesture sensor. While the final project successfully integrated the gesture sensor, the camera functionality was not implemented. For this project, I utilized an ATMEL V71 Xplained board, programming it in C using the Keil IDE (www.keil.com).
Description
Tom DesRosiers
This project served as the capstone of my graduate course, System on a Chip Studio (EEE6994), at Lawrence Technological University. The course emphasized developing skills to utilize features of a System on a Chip and interfacing with hardware peripherals using C. The majority of the coursework revolved around a final project and presentation, providing hands-on experience and practical application of the concepts learned.
The slideshow below is the presentation delivered in class.
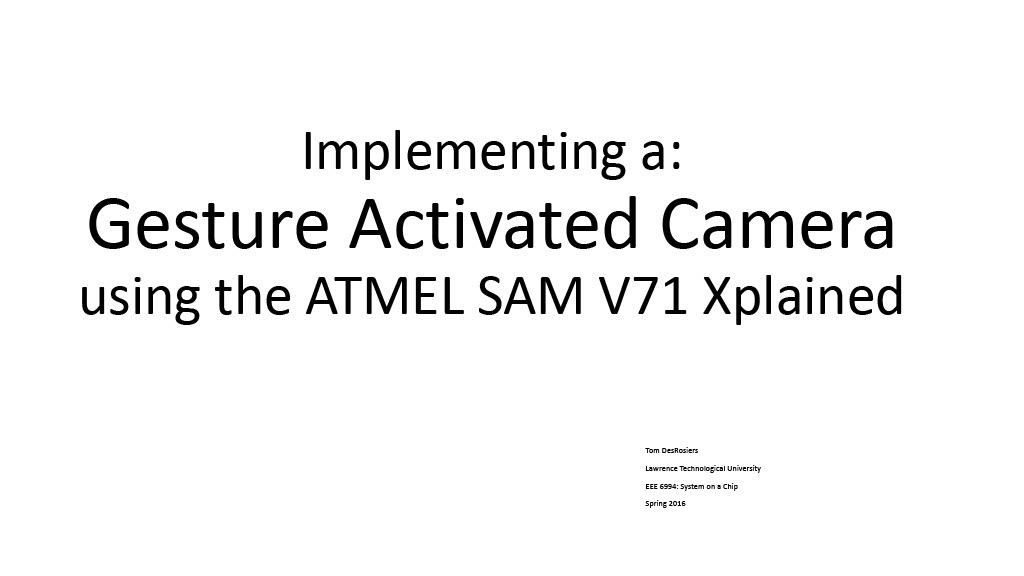
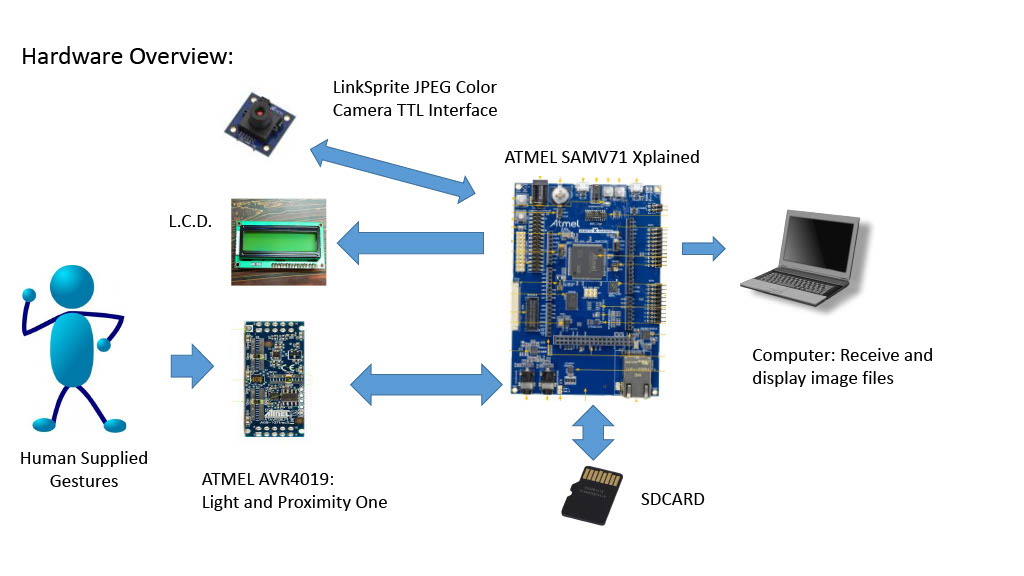
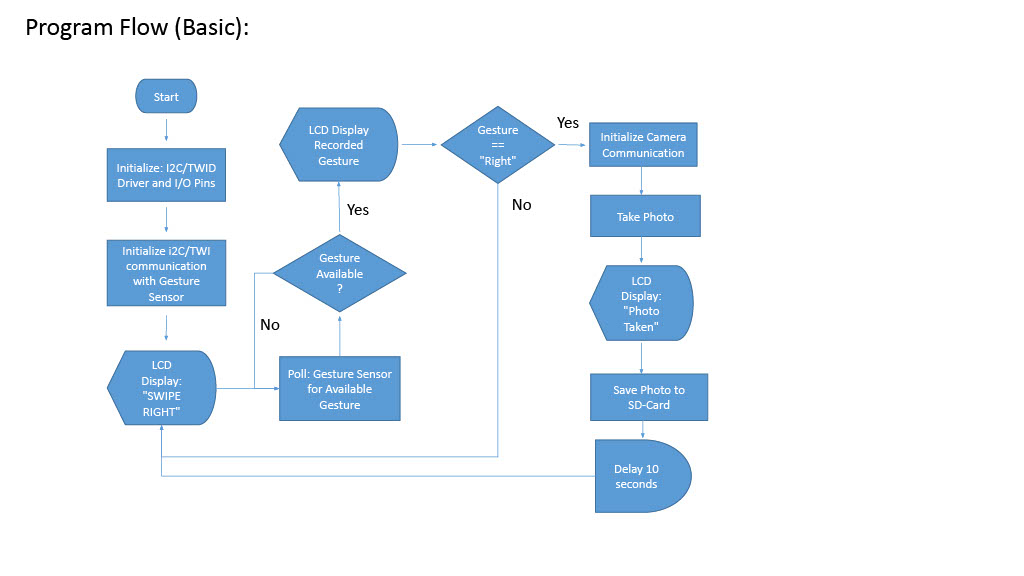
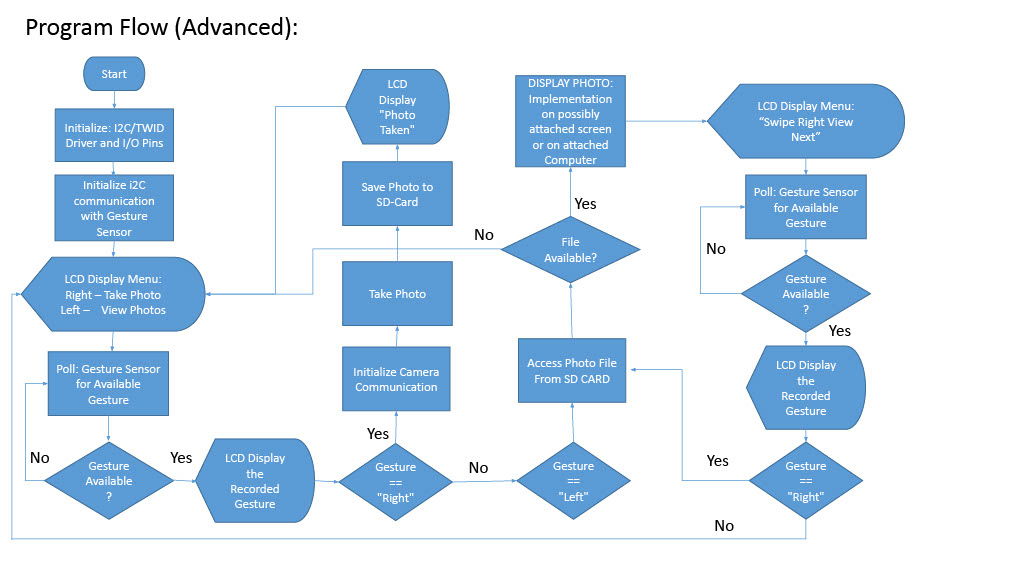
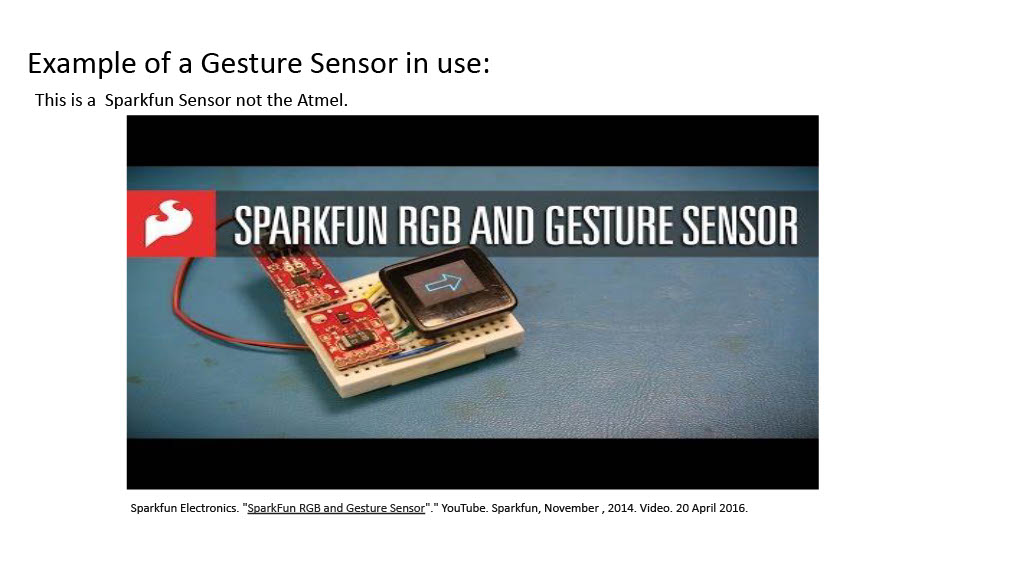
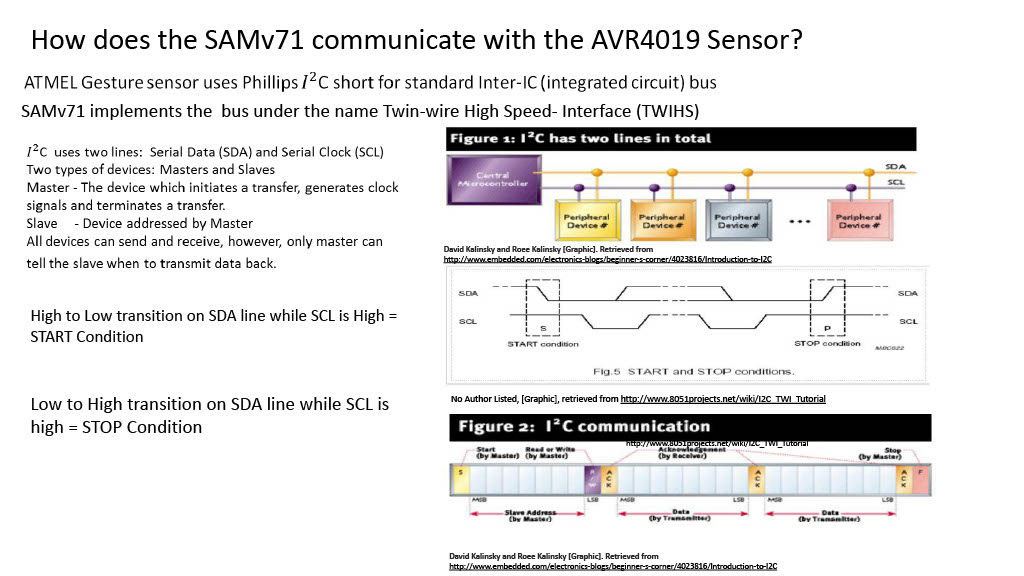
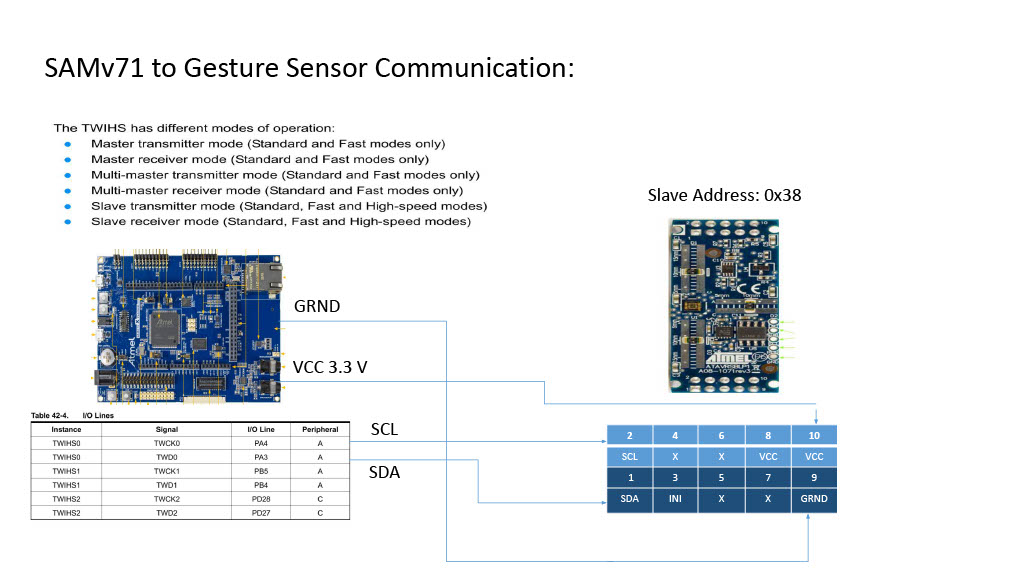
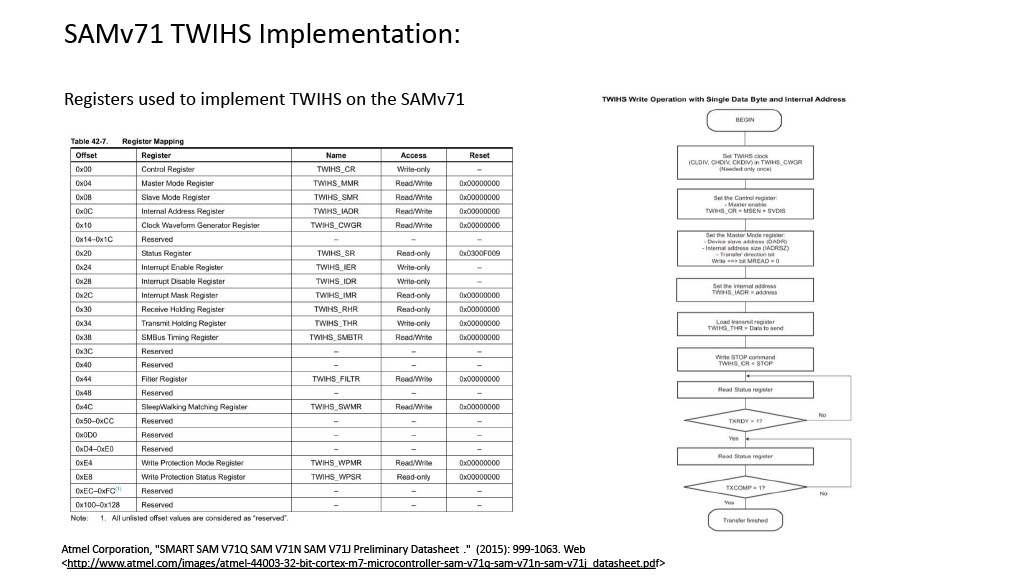
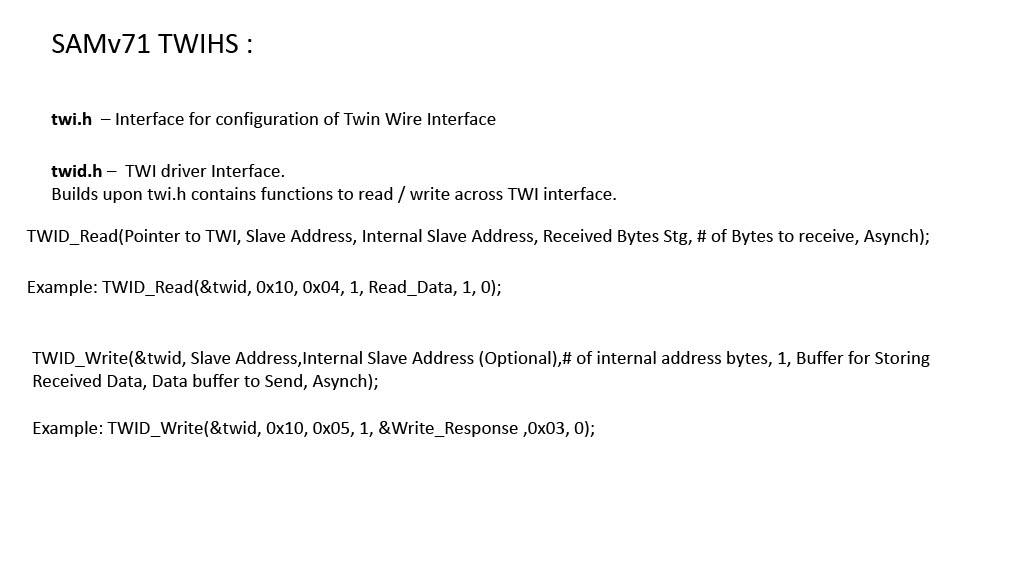
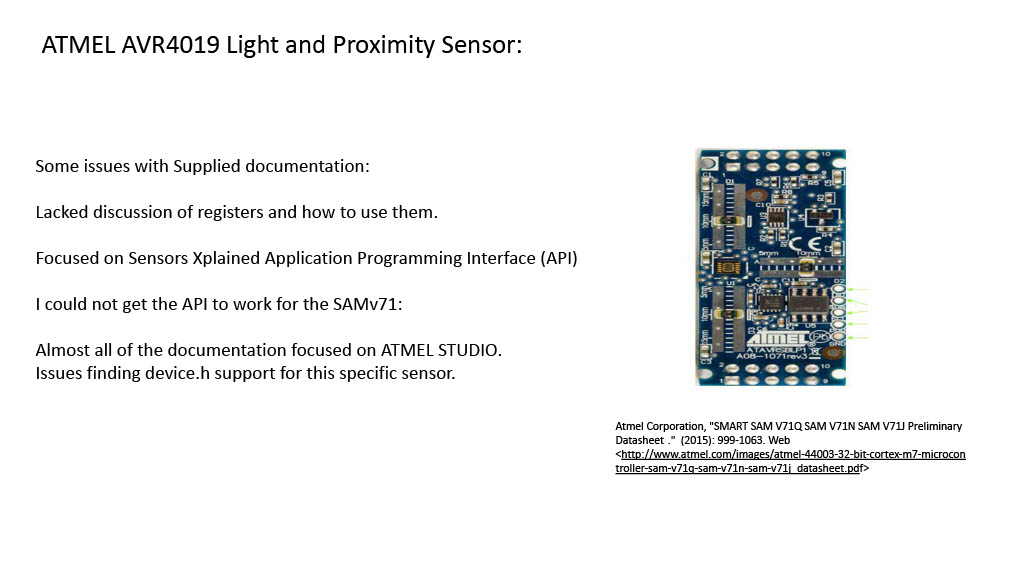
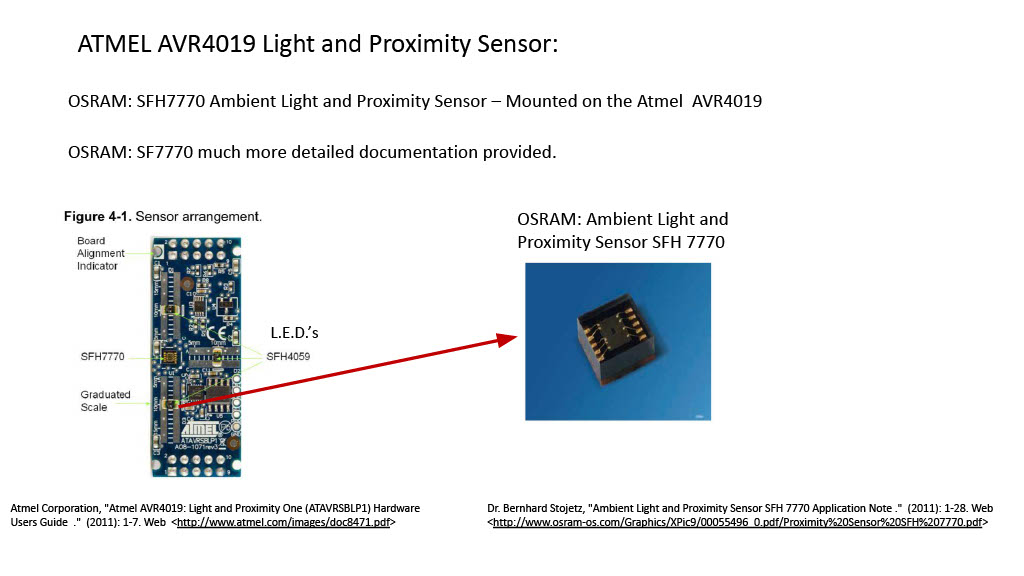
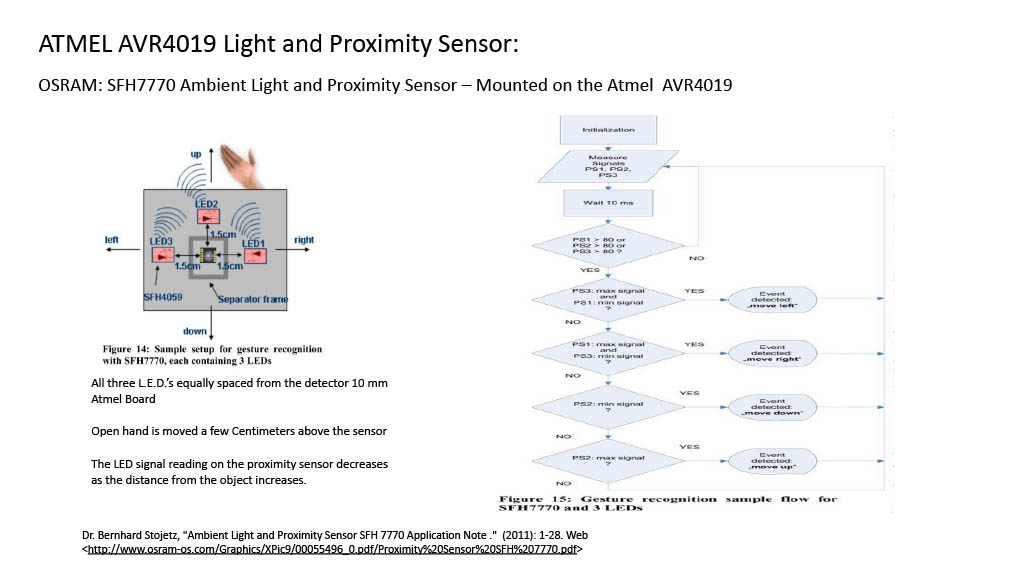
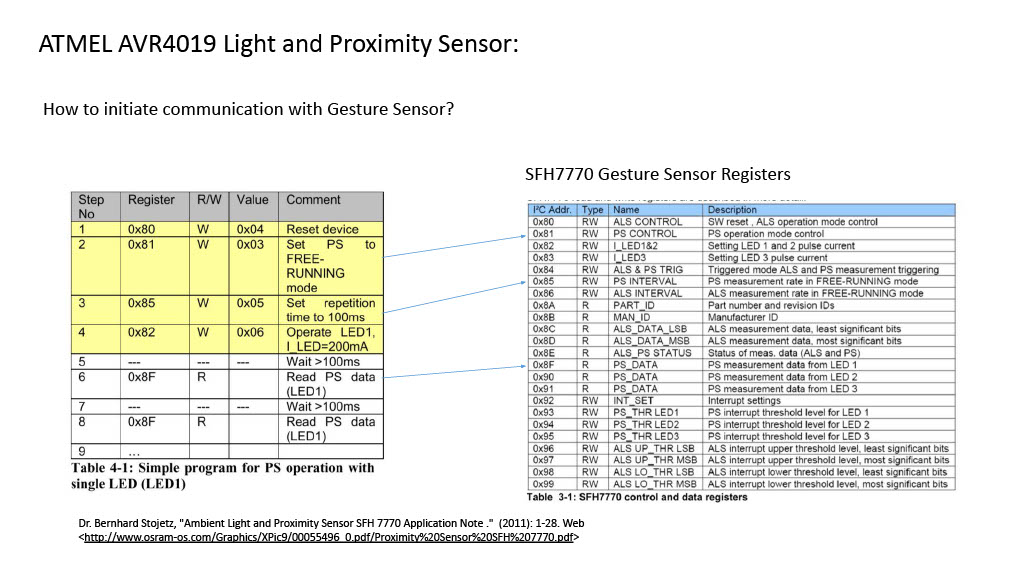
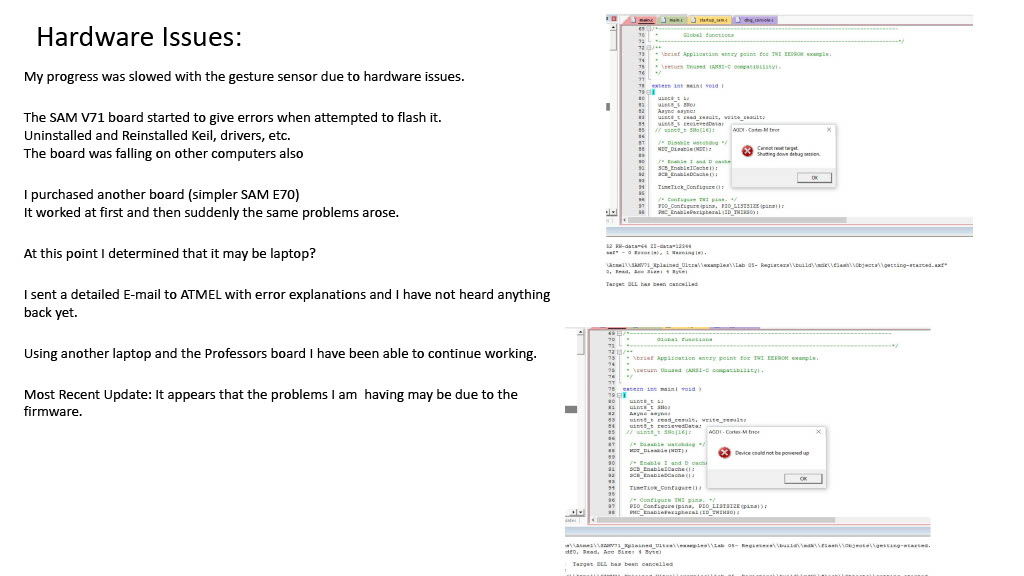
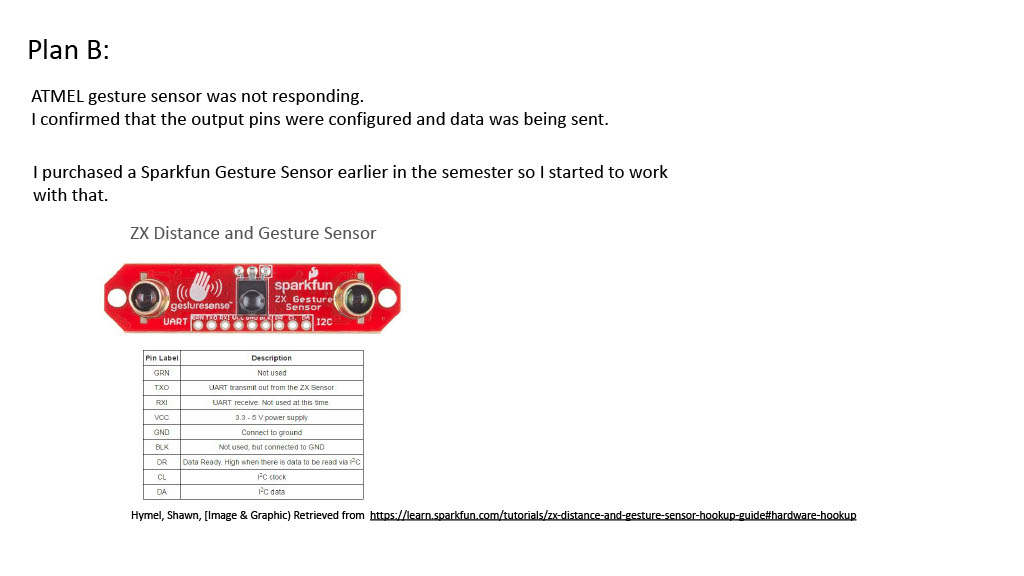
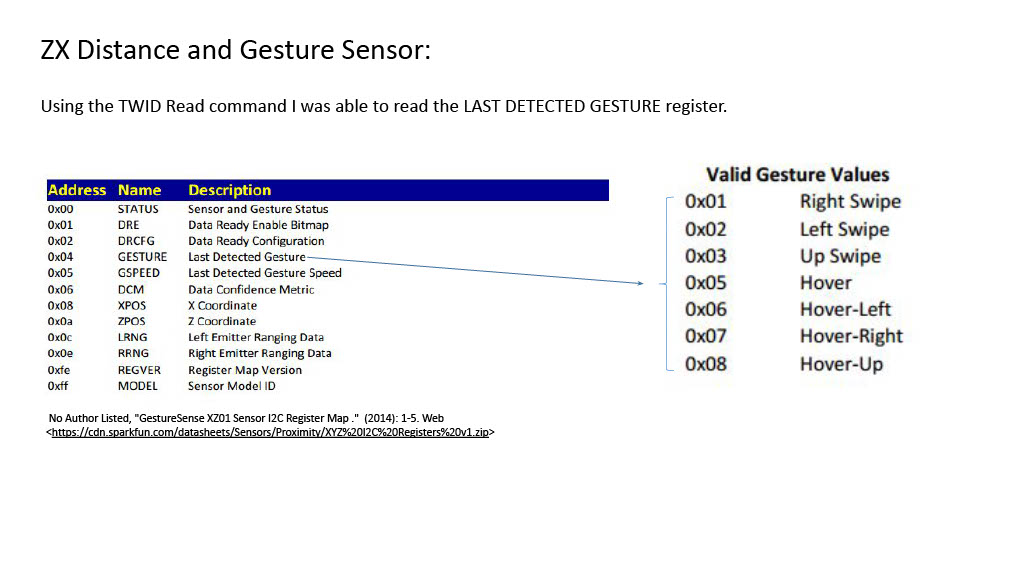
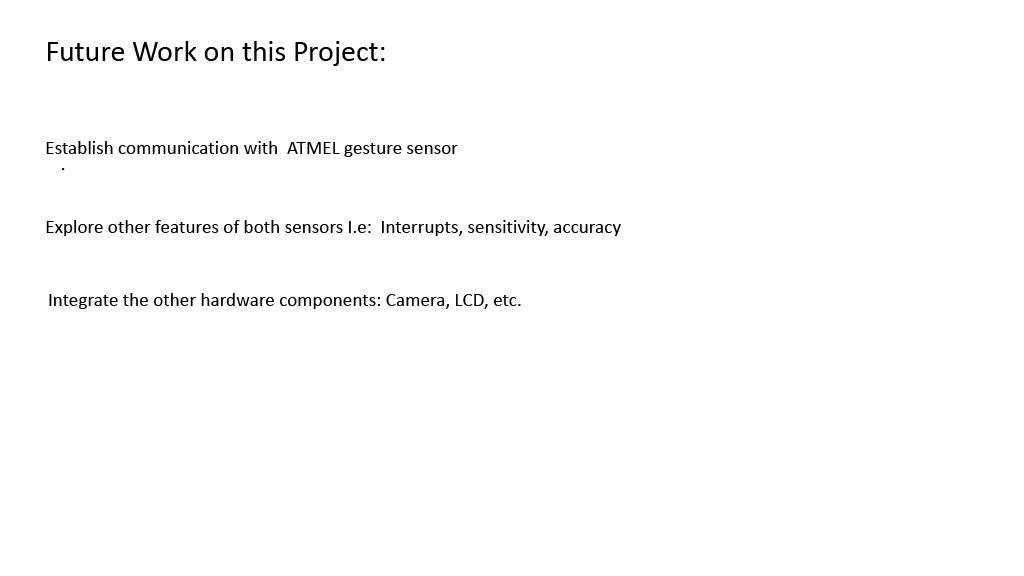
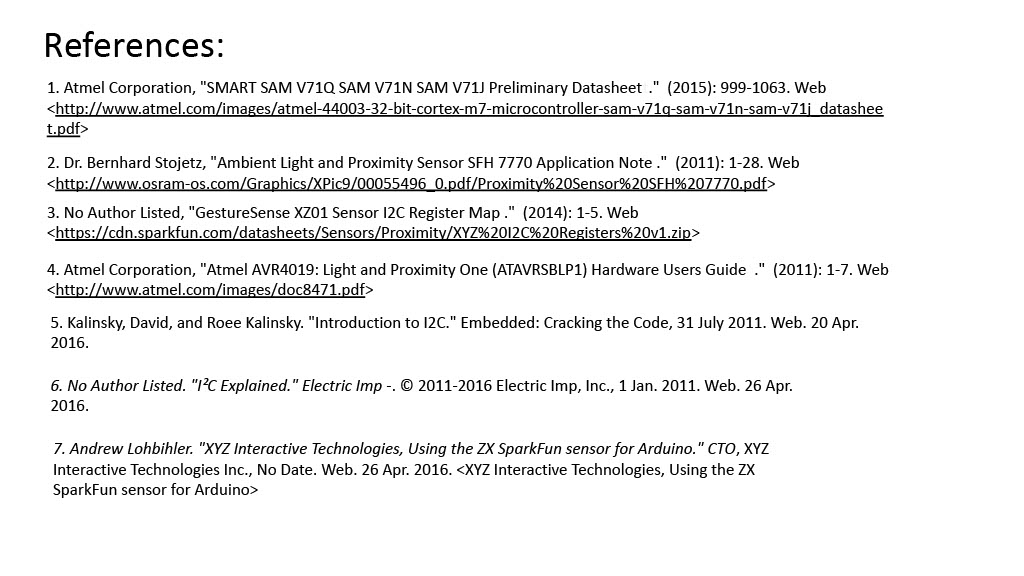
​Here is the final C code I ran using the Keil Embedded Development kit.
/*
* Tom DesRosiers
* EEE 6994: System on a Chip
* Code for Final Project
*/
#include "board.h"
#include
#include
#include
#include
#include
/*----------------------------------------------------------------------------
* Local definitions
*----------------------------------------------------------------------------*/
/** TWI clock frequency in Hz. */
#define TWCK 100000
/** Slave address of twi_eeprom example.*/
#define Gesture_Address 0x38
/** EEPROM Pins definition */
#define TWI_PINS PINS_TWI0
/** TWI0 peripheral ID for EEPROM device*/
#define BOARD_ID_TWI ID_TWIHS0
/** TWI0 base address for EEPROM device */
#define BASE_ADDRESS TWIHS0
/*----------------------------------------------------------------------------
* Local variables
*----------------------------------------------------------------------------*/
/** PIO pins to configure. */
static const Pin pins[] = TWI_PINS;
/** TWI driver instance.*/
static Twid twid;
/*----------------------------------------------------------------------------
* Global functions
*----------------------------------------------------------------------------*/
extern int main( void )
{
uint8_t i;
uint8_t SNo[16];
Async async;
uint32_t result;
uint8_t value;
//Store Write_Response
uint8_t Write_Response=0x03;
uint8_t Read_Data[10];
/* Disable watchdog */
WDT_Disable(WDT);
TimeTick_Configure();
/* Configure TWI pins. */
PIO_Configure(pins, PIO_LISTSIZE(pins));
PMC_EnablePeripheral(BOARD_ID_TWI);
/* Configure TWI */
TWI_ConfigureMaster(BASE_ADDRESS, TWCK, BOARD_MCK);
/* Initialize TWID instance */
TWID_Initialize(&twid, BASE_ADDRESS);
//Enter Loop for reading and interpreting gestures
while(1)
{
//Read the value of the Gesture Sensor Register 0x04 "Last Detected Register"
// and store in Read_Data
result=TWID_Read(&twid, 0x10, 0x04, 1, Read_Data, 1, 0);
value = Read_Data[0];
//Check the value of the gesture register and compare to display appropriate gesture
switch(value){
case 0x01:
{
printf("Right Swipe\n \r ");
break;
}
case 0x02:
{
printf("Left Swipe\n \r ");
break;
}
case 0x03:
{
printf("Up Swipe\n \r ");
break;
}
case 0x05:
{
printf("Hover \n \r");
break;
}
case 0x06:
{
printf("Hover-Left \n \r");
break;
}
case 0x07:
{
printf("Hover-Right \n \r");
break;
}
case 0x08:
{
printf("Hover-up \n \r ");
break;
}
default:
{
printf("None \n \r ");
}
//Pause 100 milliseconds
Wait(100);
}
} // End Loop
} // End Main